Introduction
Powerful workflow editor builder for sequential workflows. Written in TypeScript. Mainly designed to work with the Sequential Workflow Designer component. To execute your model you may use the Sequential Workflow Machine or any other workflow engine. It supports front-end and back-end strict validation of the model. 0 external dependencies.
Check our online examples.
Why I Need It?
Before you start working with the workflow you need to define your workflow model: what a user can do with your no-code/low-code application. At the highest level you need to define steps. Each step does some specific task, so each step requires some specific configuration. This configuration in the Sequential Workflow Designer component is available via editors. Of course you can manually create editors from code and this approach is quite popular. But the main problem here is that how to validate the workflow definition outside the editor? For example: how to validate the definition in the back-end? This problem can be solved by extracting the validation from editors and moving it to the separate layer.
There are many other problems to solve like: cross validation of configuration fields, dynamic values, single source of truth for default values, etc.
This is a reason why we've created the Sequential Workflow Editor. Our component provides a split architecture and a subset of good practices, that allows you to create no-code/low-code application with high maintainable code.
Main Concept
The Sequential Workflow Editor is divided into three parts: the definition model, the editor generator, and the definition validator. The model essentially describes how the editor should be generated for each step or for the root of the definition. This description is used to create the editor in the browser and also to validate the definition in the backend or other places outside the designer's interface. The description includes not only a static definition of the fields but also a set of rules and constraints that are used to validate the definition. What is important sometimes constraints are described by JavaScript functions, so you can use the full power of JavaScript to validate your definition.
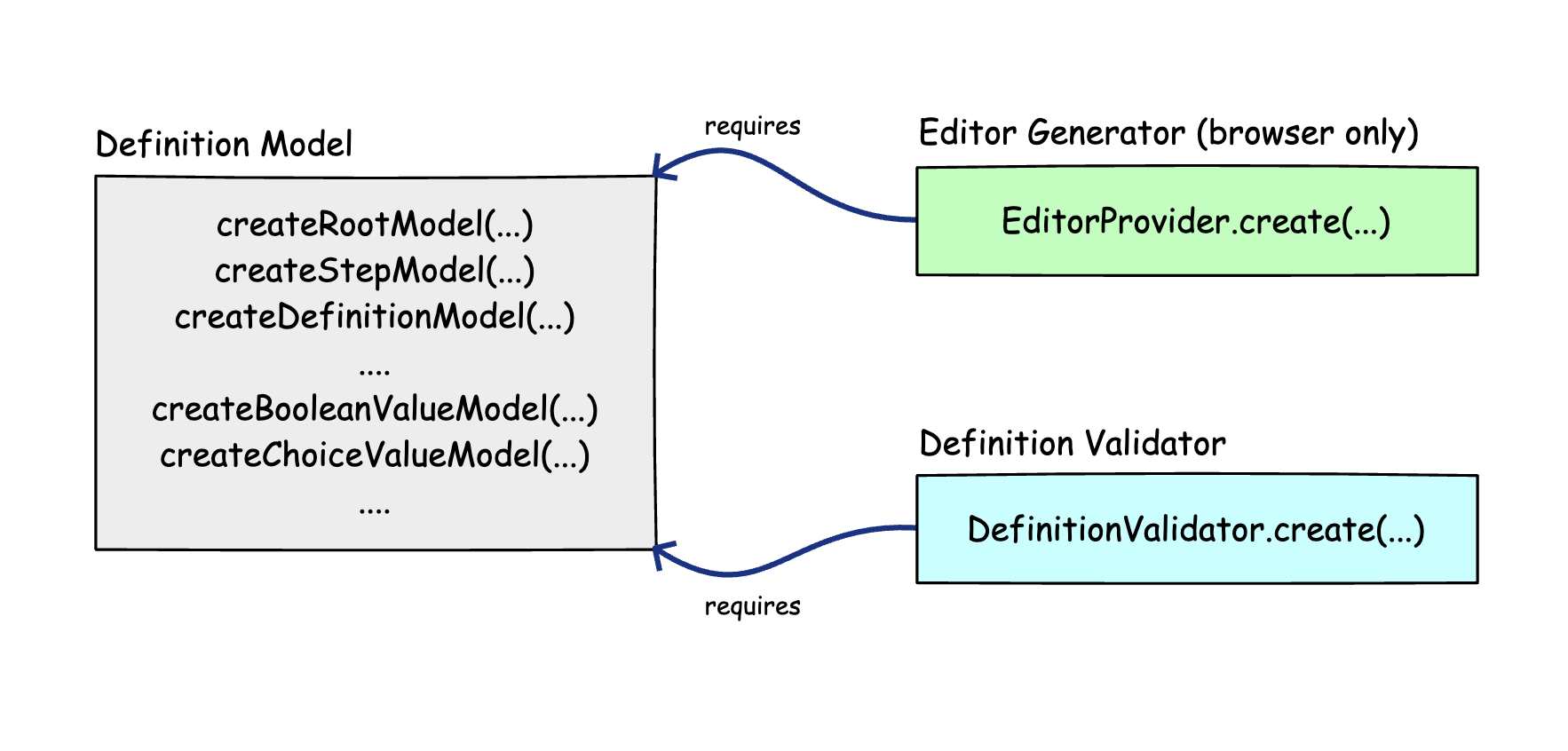
Let's continue this introduction with a simple example. Let's consider a simple step in the workflow that sends an email. The interface of this step can look like this:
import { Step } from 'sequential-workflow-model';
export interface SendEmailStep extends Step {
type: 'sendEmail';
componentType: 'task';
properties: {
to: string;
addFooter: boolean;
};
}
We have two fields here: to
and addFooter
. The to
field keeps the email address of the recipient and the addFooter
field is a boolean flag that indicates whether to add a footer to the email. To build an editor for this step we need two inputs, one for the email address and one for the boolean flag. If we would implement this editor manually, we would need to write a lot of code to create the editor and to validate the definition. But with the Sequential Workflow Editor we can describe the step in a declarative way and the editor will be generated automatically.
import { createStepModel } from 'sequential-workflow-editor-model';
export const sendEmailStepModel = createStepModel<SendEmailStep>('sendEmail', 'task', step => {
step.property('to').value(
createStringValueModel({})
);
step.property('addFooter').value(
createBooleanValueModel({})
);
});
This is enough to generate the editor for the step.
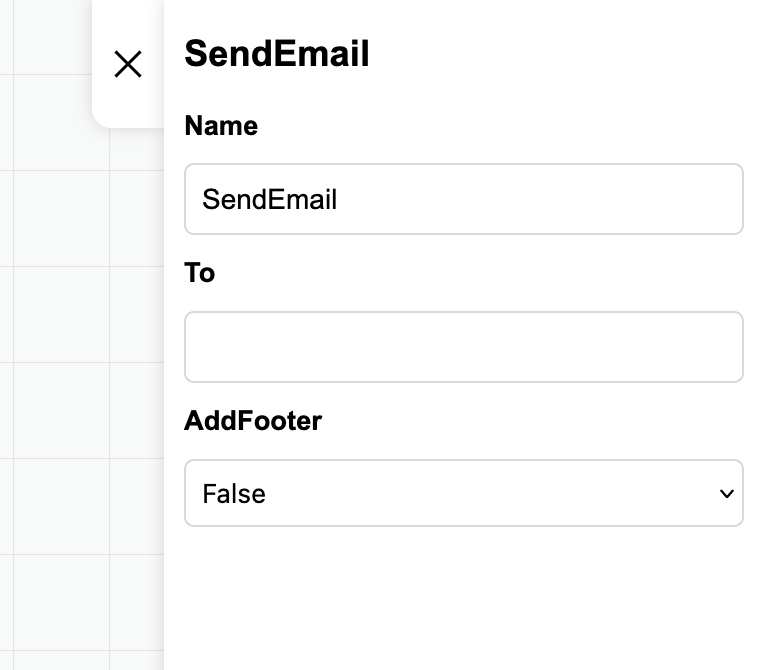
After a few seconds we will notice that, we need to add some constraints to the fields, because we expect specific data from the user, not just any data. For example, the to
field should be a valid email address, not shorter than 5 characters.
step.property('to').value(
createStringValueModel({
minLength: 5,
pattern: /^\S+@\S+\.\S+$/
})
);
The final effect looks like this:
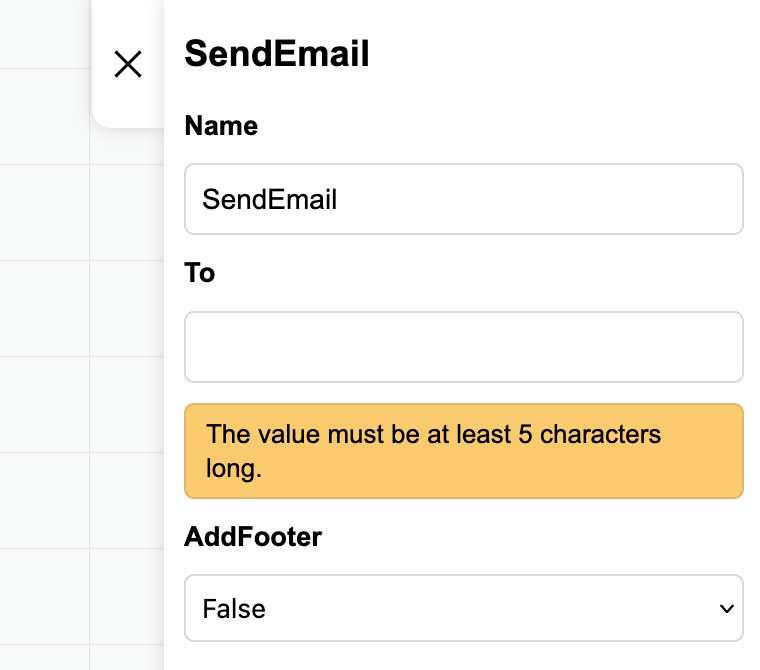
The most important part here is that, the created model is not only used to generate the editor, but also to validate the definition. The same constraints that are used to generate the editor are also used to validate the definition for example in the back-end. This gives us a single source of truth for the definition and its constraints.
const validator = DefinitionValidator.create(model, ...);
const error = validator.validate(definition);
The Sequential Workflow Editor supports a lot of different field types and constraints. More details you can find in this documentation.
Check our online examples to see how to the Sequential Workflow Editor is working in practice.